Explore Quadratic’s three pillars
AI
Understand any data set using natural language with a built-in AI assistant that will do things for you — analyze data, write code, and more.
Learn more
2Code
Learn how to leverage Python and JavaScript natively in your spreadsheet alongside traditional formulas.
Code 101
3Connections
Connect directly to databases, data warehouses, and APIs to execute queries, analyze results, and visualize insights.
See all connections
Start with a template
Explore what’s possible in a Quadratic spreadsheet without starting from scratch.
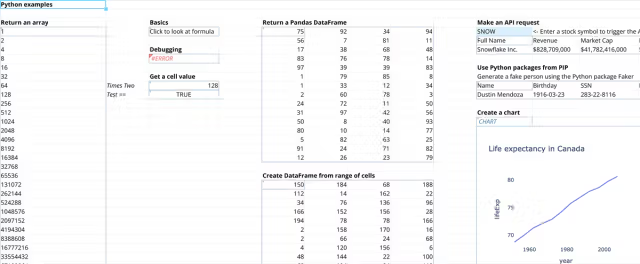
Python Intro Template
Get started with writing Python in Quadratic.
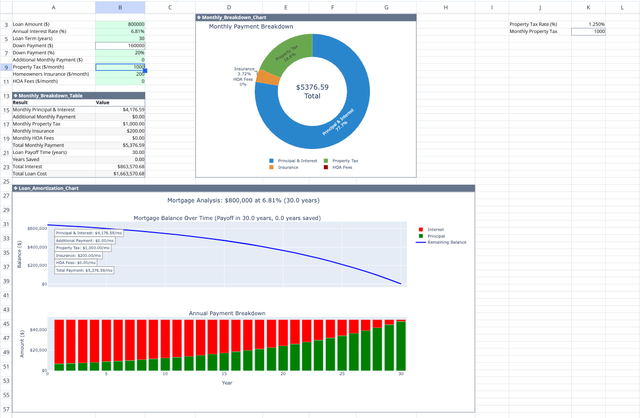
Pay Off Mortgage Faster Calculator
Visualize your path to home ownership freedom with early payments.
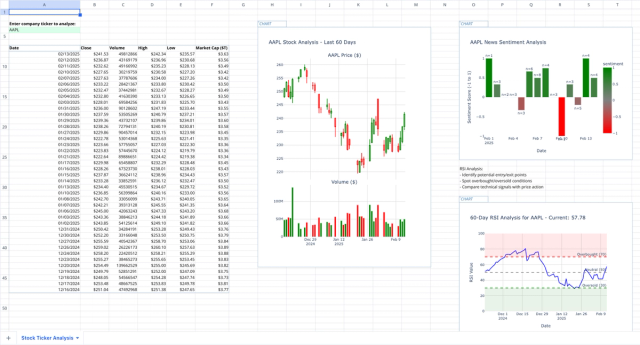
Stock Market Analysis Template
Study the markets with an interactive spreadsheet tracking any publicly traded company.
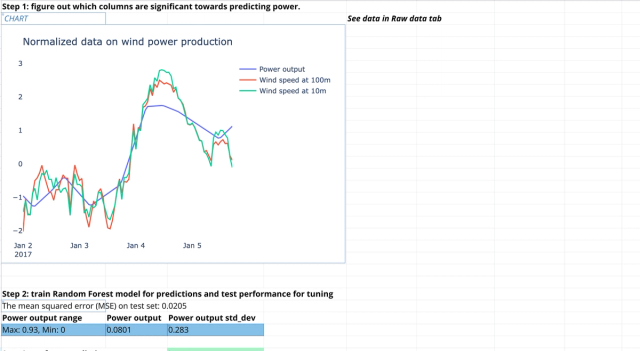
Machine Learning Tutorial Template
Build your first scikit-learn example using renewable energy data from a wind farm.
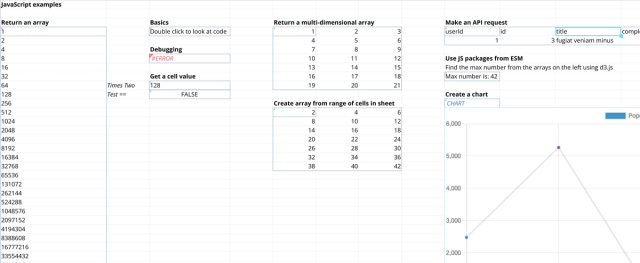
JavaScript Intro Template
Get started with writing JavaScript in Quadratic.
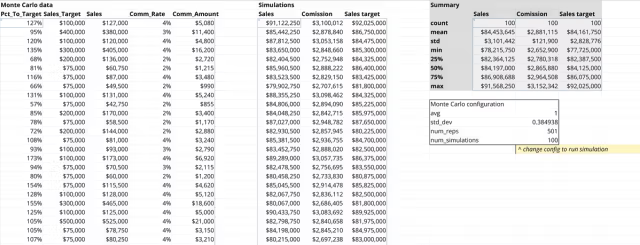
Monte Carlo Simulation Template
Work with large simulation data using Monte Carlo.